There is a new version of this book. If you want to get an electronic copy or a paperback, see Using Raku for more details.
100 Programming Challenges Solved with the Perl 6 Programming Language
This book is a collection of different programming challenges and solutions in Perl 6. It can be used as an exercise book, when you are learning Perl 6, or as a reference book when you are teaching it.
It is assumed that the reader knows thebasics of Perl 6 and wants to have some practice.
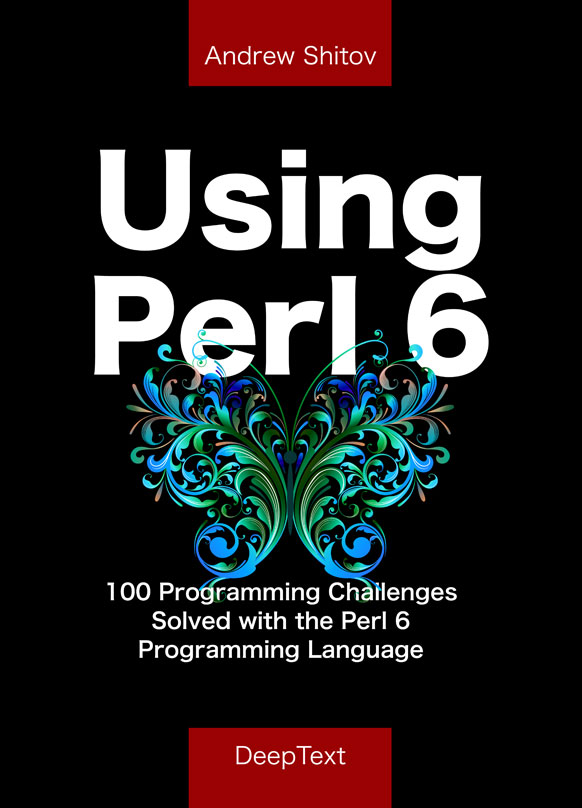
Andrew Shitov. Using Perl 6
© Andrew Shitov, author, 1 November 2017
Published on perl6.online in September 2019
Published by DeepText, Amsterdam
www.deeptext.media
ISBN 978-90-821568-1-2
Navigate to the contents below or download the PDF version.
Book Contents
Introduction
Part 1
Chapter 1. Strings
1.1. Using strings
1.2. Modifying string data
6. Removing blanks from a string
7. Camel case
11. Caesar cipher
1.3. Text analysis
12. Plural endings
14. The longest common substring
15. Anagram test
16. Palindrome test
Chapter 2. Numbers
2.1. Using numbers
19. π
20. Factorial!
22. Print squares
23. Powers of two
25. Compare numbers approximately
27. Prime numbers
29. Prime factors
31. Divide by zero
2.2. Random numbers
33. Neumann’s random generator
34. Histogram of random numbers
2.3. Mathematical problems
35. Distance between two points
2.4. Numbers and strings
39. Unicode digits
40. Guess the number
42. Integer as binary, octal, and hex
43. Sum of digits
44. Bit counter
45. Compose the largest number
47. Spelling numbers
Chapter 3. Aggregate Data Types
3.1. Manipulating lists and arrays
48. Swap two values
49. Reverse a list
50. Rotate a list
52. Incrementing array elements
3.2. Information retrieval
55. Sum of the elements of an array
57. Moving average
59. First odd number
61. Number of occurrences in array
3.3. Working with subroutines
65. Passing arrays to subroutines
66. Variadic parameters in a sub
3.4. Multi-dimensional data
70. Product table
71. Pascal triangle
Chapter 4. Regexes and Grammars
4.1. Regex matching
73. Count words
74. Skipping Pod documentation
4.2. Substitutions with regexes
78. Separate digits and letters
81. Pig Latin
4.3. Using grammars
86. Basic calculator
Part 2
Chapter 5. Date and Time
88. Formatted date
90. Leap years
Chapter 6. Parallel Computing
91. Setting timeouts
92. Sleep Sort
Chapter 7. Miscellaneous
95. The cat
utility
96. The uniq
utility
99. Morse to text
Keep experimenting and make your way in running the Perl 6 code in production applications!
The book was published in the end of 2017 in paper and later as e-book, and was published online at perl6.online in the end of 2019.
The content on this page is free of charge and may be used for any educational purposes. See more books from me at deeptext.media.